- Java Reader For Mac Advantage Load Balancer
- Java Reader For Mac Advantage Load Windows 7
- Java Reader For Mac Advantage Load Windows 10
Java Reader For Mac Advantage Load Balancer
We would like to show you a description here but the site won’t allow us.
The JavaReader class, java.io.Reader
, is the base class for all Reader
subclasses in the Java IO API. A Java Reader
is like a Java InputStream except that it is character based rather than byte based. In other words, a Java Reader
is intended for reading text (characters), whereas an InputStream
is intended for reading raw bytes.
- Java FileReader Class for beginners and professionals with examples on Java IO or Input Output in Java with input stream, output stream, reader and writer class. The java.io package provides api to reading and writing data.
- Advanced IP Scanner. Reliable and free network scanner to analyse LAN. The program shows all network devices, gives you access to shared folders, provides remote control of computers (via RDP and Radmin), and can even remotely switch computers off.
- The Java.io.Reader class is a abstract class for reading character streams. Class declaration. Following is the declaration for Java.io.Reader class − public class Reader extends Object implements DataOutput, DataInput, Closeable Field. Following are the fields for Java.io.Reader class −.
Readers and Sources
A Reader
is typically connected to some source of data like a file, char array, network socket etc. This is also explained in more detail in the Java IO Overview text.

/https%3A%2F%2Ftechnofaq.org%2Fwp-content%2Fuploads%2F2016%2F02%2Fdisk-drill-07.jpeg)
Characters in Unicode
Today, many applications use Unicode (UTF-8 or UTF-16) to store text data. It may take one or more bytes to represent a single character in UTF-8. In UTF-16 each character takes 2 bytes to represent. Therefore, when reading text data, a single byte in the data may not correspond to one character in UTF. If you just read one byte at a time of UTF-8 data via an InputStream
and try to convert each byte into a char
, you may not end up with the text you expected.
To solve this problem we have the Reader
class. The Reader
class is capable of decoding bytes into characters. You need to tell the Reader
what character set to decode. This is done when you instantiate the Reader
(actually, when you instantiate one of its subclasses).
Java Reader Subclasses
You will normally use a Reader
subclass rather than a Reader
directly. Java IO contains a lot of Reader
subclasses. Here is a list of the Java Reader
subclasses:
Here is an example of creating a Java FileReader
which is a subclass of Java Reader
:
Read Characters From a Reader
The read()
method of a Java Reader
returns an int which contains the char
value of the next character read. If the read()
method returns -1, there is no more data to read in the Reader
, and it can be closed. That is, -1 as int value, not -1 as byte or char value. There is a difference here!
Here is an example of reading all characters from a Java Reader
:
Notice how the code example first reads a single character from the Java Reader
and checks if the char numerical value is equal to -1. If not, it processes that char
and continues reading until -1 is returned from the Reader
read()
method.
Read Array of Characters From Reader
The Java Reader
class also has a read()
method that takes a char
array as parameter, as well as a start offset and length. The char
array is where the read()
method will read the characters into. The offset parameter is where in the char
array the read()
method should start reading into. The length parameter is how many characters the read()
method should read into the char
array from the offset and forward. Here is an example of reading an array of characters into a char
array with a Java Reader
:
The read(char[], offset, length)
method returns the number of characters read into the char
array, or -1 if there are no more characters to read in the Reader
, for instance if the end of the file the Reader
is connected to has been reached.
Read Performance
Reading an array of characters at a time is faster than reading a single character at a time from a Java Reader
. The difference can easily be a factor 10 or more in performance increase, by reading an array of characters rather than reading a single character at a time.
The exact speedup gained depends on the size of the char
array you read, and the OS, hardware etc. of the computer you are running the code on. You should study the hard disk buffer sizes etc. of the target system before deciding. However buffer sizes of 8KB and up will give a good speedup. However, once your char
array exceeds the capacity of the underlying OS and hardware, you won't get a bigger speedup from a bigger char
array.
You will probably have to experiment with different byte array size and measure read performance, to find the optimal char
array size.
Transparent Buffering via BufferedReader
You can add transparent, automatic reading and buffering of an array of bytes from a Reader
using a Java BufferedReader . The BufferedReader
reads a chunk of chars
into a char
array from the underlying Reader
. You can then read the bytes one by one from the BufferedReader
and still get a lot of the speedup that comes from reading an array of chars
rather than one character at a time. Here is an example of wrapping a Java Reader
in a BufferedReader
:
Notice, that a BufferedReader
is a Reader
subclass and can be used in any place where an Reader
can be used.
Skip Characters
The Java Reader
class has a method named skip()
which can be used to skip over a number of characters in the input that you do not want to read. You pass the number of characters to skip as parameter to the skip()
method. Here is an example of skipping characters from a Java Reader
:
This example tells the Java Reader
to skip over the next 24 characters in the Reader
. The skip()
method returns the actual number of characters skipped. In most cases that will be the same number as you requested skipped, but in case there are less characters left in the Reader
than the number you request skipped, the returned number of skipped characters can be less than the number of characters you requested skipped.
Closing a Reader
When you are finished reading characters from a Reader
you should remember to close it. Closing an Reader
is done by calling its close()
method. Here is how closing an Reader
looks:
You can also use the Java try with resources construct introduced in Java 7. Here is how to use and close a InputStreamReader
looks with the try-with-resources construct:
Notice how there is no longer any explicit close()
method call. The try-with-resources construct takes care of that.
- Java.io package classes
- Java.io package extras
- Java.io package Useful Resources
- Selected Reading
Introduction
The Java.io.PushbackReader class is a character-stream reader that allows characters to be pushed back into the stream.
Class declaration
Following is the declaration for Java.io.PushbackReader class −
Field
Following are the fields for Java.io.PushbackReader class −
Java Reader For Mac Advantage Load Windows 7
protected Reader in − This is the character-input stream.
protected Object lock − This is the object used to synchronize operations on this stream.
Class constructors
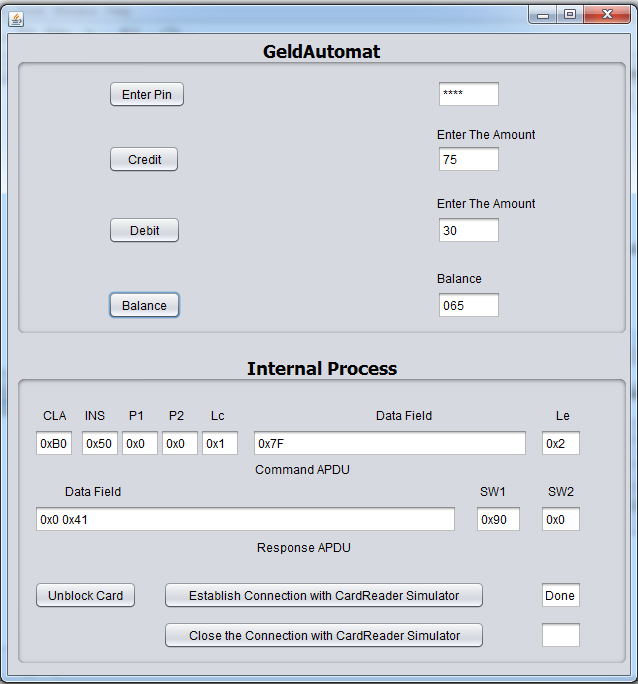
Sr.No. | Constructor & Description |
---|---|
1 | PushbackReader(Reader in) This creates a new pushback reader with a one-character pushback buffer. |
2 | PushbackReader(Reader in, int size) This creates a new pushback reader with a pushback buffer of the given size. |
Java Reader For Mac Advantage Load Windows 10
Class methods
Sr.No. | Method & Description |
---|---|
1 | void close() This method closes the stream and releases any system resources associated with it. |
2 | void mark(int readAheadLimit) This method marks the present position in the stream. |
3 | boolean markSupported() This method tells whether this stream supports the mark() operation, which it does not. |
4 | int read() This method reads a single character. |
5 | int read(char[] cbuf, int off, int len) This method reads characters into a portion of an array. |
6 | boolean ready() This method tells whether this stream is ready to be read. |
7 | void reset() This method resets the stream. |
8 | long skip(long n) This method skips characters. |
9 | void unread(char[] cbuf) This method pushes back an array of characters by copying it to the front of the pushback buffer. |
10 | void unread(char[] cbuf, int off, int len) This method pushes back a portion of an array of characters by copying it to the front of the pushback buffer. |
11 | void unread(int c) This method pushes back a single character by copying it to the front of the pushback buffer. |
Methods inherited
This class inherits methods from the following classes −
- Java.io.Reader
- Java.io.Object
