- Exciting New Features For Iphone 11
- Exciting New Features & New Plans 12x16
- Exciting New Features & New Plans Floor Plans
- Exciting New Features For Small
- Exciting New Features For Ipad
- Exciting New Features & New Plans One Story
MariaDB 10.5 was released in June 2020 and it will be supported until June 2025. This is the current stable version and comes with more exciting new features. In this blog, I am going to explain the new and exciting features involved in MariaDB 10.5. Microsoft Deals Blow With A Bunch Of Exciting New Edge Features January 24, 2021 January 24, 2021 Swaira Shabir 0 Comments January 24, 2021 Swaira Shabir 0 Comments.
Perhaps the most cited reason for not using Java for development is that it’s too verbose. Developers complain that there’s too much boilerplate, and that no new features for Java developers have been released in a long time. The idea that Java is not growing and changing is flatly false. Although it’s true that other languages built on the JVM have gained significant traction, especially Kotlin, Java is certainly fighting back. These new Java features are coming… and fast.
Since Oracle has split JDK into a Commercial Oracle JDK and OpenJDK, the development and release cycle for the JDK has increased at an alarming rate. On OpenJDK’s website, you can see the last 5 versions of the OpenJDK have all been released since 2018. The first 9 versions of Java SE were released over the course of 18 years.
Project Amber
The goal of Project Amber is to add productivity-oriented features to the Java language. Project Amber is taking huge steps forward in the developer experience in Java. As someone with a habit of furtively eyeing other languages, I felt a sense of relief and excitement knowing the direction OpenJDK was taking Java. Here are the main goals of project Amber, per the OpenJDK Website.
Delivered:
- JEP 286 Local-Variable Type Inference (
var
) (JDK 10) - JEP 323 Local-Variable Syntax for Lambda Parameters (JDK 11)
- JEP 361 Switch Expressions (JDK 14)
Currently in progress:
- JEP 360 Sealed Types (Preview)
- JEP 359 Records (Second preview)
- JEP 368 Text Blocks (Second Preview)
- JEP 375 Pattern Matching for
instanceof
(Second Preview)
Quite a few features, and each and every one represents a significant change to the way you will write Java code in the future.
The var
Keyword (JDK 10)
The var
keyword is a new way to declare variables using type inference. var
allows you to declare a variable without specifying it’s type. In Java, this goes against the grain of always having to define the type of a variable. There is some dissent among professionals if var
should be embraced or avoided due to conventional Java’s insistence on type specification.
In the right environment, I think a style guide which includes the use of var
, where appropriate, would allow for significant readability improvements. The var
keyword performs best in small, self-explanatory methods where types can be omitted without losing clarity of what a variable is.
var
OpenJDK provides principles and Style Guidelines to help you determine when to use var
:
- Reading code is more important than writing code.
- Code should be clear from local reasoning.
- Code readability shouldn’t depend on IDEs.
- Explicit types are a tradeoff.
If your code is aligned with these principles when var
is used, then you can safely use it. If not, you should default back to explicitly typing your variables. Keep in mind that var
is only available for local variables.
JEP 323, Local-Variable Syntax for Lambda Parameters (JDK 11) is an extension of the var
keyword to also be usable in Lambda parameters.
var
keyword in lambda parametersThis simply enables you to use annotations with lambda parameters without requiring you to explicitly define the type of the lambda parameter.
switch
Expressions (JDK 14)
The switch
expression is a new type of switch
which allows you to easily assign the result of the switch computation to a variable.
switch
expressionThis alleviates the requirement of having to use return
or break
to exit a switch statement. This also allows for simpler variable assignment, without having to repeat result = x + y; break;
, result = x * y; break;
, etc.
In short, switch
expressions make switch
es shorter, easier to read, and less error prone.
Pattern Matching for instanceof
(JDK 15, Second Preview)
JEP 375 will give Java’s instanceof
test some much-needed love. Every Java programmer is familiar with the following for checking the type of an object, and then retrieving the value of a field from it.
instanceof
check, cast, and use of an unknown Object
.The above is verbose and unnecessary. Most of the time you do an instanceof
test, you will be casting the object to that type and performing some type-specific operation on it. Pattern matching will make this more concise and remove the cast entirely.
This becomes especially annoying when you need to test for multiple different types, as in the below example by Gavin Bierman and Brian Goetz.
Using pattern matching and switch
expressions together, the above 17 lines of code, which is 66% boilerplate, could be simplified to just 8 lines.
The ability to use pattern matching with switch
is not planned for the same release as the regular pattern matching, but I couldn’t help but include it. The clarity and brevity of the above code is impressive – especially for Java!
Text Blocks (JDK 15, Second Preview)
Text blocks are a simple quality of life enhancement for writing longer strings in Java. Whether you want to embed a block of code from another language, an HTML snippet, or a JSON payload, text blocks will simplify this:
To this:
Text blocks support standard escape sequences and are java.lang.String
s.
Records (JDK 15, Second Preview)
Java Records are immutable classes that can be specified using special, compact syntax. If you are familiar with Lombok, records are similar to classes annotated with @Value
, they are data holders that have the following properties (modified for readability from JEP 359):
- A private final field for each component of the state description;
- A public getter for each field in the state description;
- A public constructor which initializes each field from the corresponding argument;
- Implementations of
equals
andhashCode
that say two records are equal if they are of the same type and contain the same state; and - An implementation of
toString
that includes the string representation of all the record components, with their names.
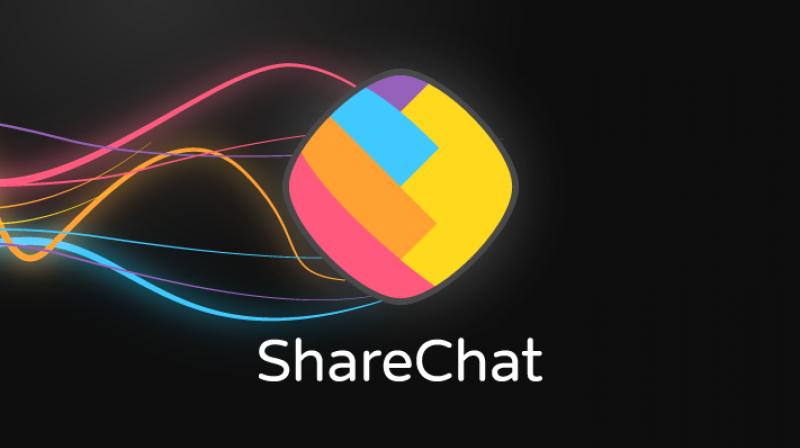
Like enum
, record
s are created using the keyword record
and a straightforward constructor-like syntax called a “state description”. Once the state description is defined, we get all 5 features above for free. It is possible to add validation in the constructor and still not be required to specify the constructor arguments or fields they will be assigned to. Instance methods are also supported. Setters are not provided, the generated fields x
and y
below are final
.
Range
record
using state description. Records are by far the most exciting new Java feature of the bunch. Records will change the way we interact with data holder classes on a day-to-day basis. I suspect that Java programmers, once beginning to use record
, will wonder how we ever did this before.
Sealed Types (JDK 15, Preview)
A sealed type is a type with restrictions placed on what can subclass it. The restrictions are defined in the type definition. My first question with this feature was, why? Why not always allow for a type to be subclassed? This was explained best in JEP 360:
In Java, a class hierarchy enables the reuse of code via inheritance: The methods of a superclass can be inherited (and thus reused) by many subclasses. However, the purpose of a class hierarchy is not always to reuse code. Sometimes, its purpose is to model the various possibilities that exist in a domain, such as the kinds of shapes supported by a graphics library or the kinds of loans supported by a financial application. When the class hierarchy is used in this way, restricting the set of subclasses can streamline the modeling.
Brian Goetz, JEP 360, 2020Sealed types allow you to specify not just what the supertype is, but also what the subtypes can be. As mentioned above, sealing allows for a special kind of semantic to be defined in a supertype. Another benefit is that the compiler is subsequently allowed to make assumptions about the subtypes, such as in our pattern matching switch expression example above – if the supertype is a sealed type, then the compiler could allow you to omit the default
clause in a switch
, as in the below example from Data Classes and Sealed Types for Java, by Brian Goetz (2019).
switch
expression example from Data Classes and Sealed Types for Java, by Brian Goetz (2019)Contribute to OpenJDK
With all the new Java features coming to the JDK in the near future, I am excited to see what the OpenJDK team dreams up next. Clearly, the developer experience is at the forefront of the great minds working on the JDK. As Java developers, we are all part of the ecosystem that sustains and improves Java. If you have an interest in contributing or providing feedback on the preview features, become a contributor to OpenJDK here.
Exciting New Features For Iphone 11
Apple recently released iOS 14.2 and iPadOS 14.2. The updates are chockfull of new features, including a few that integrate with the company's HomePod smart speakers. This second major update to iOS 14 and iPadOS 14 was released two weeks after the rollout of iOS 14.1. Continue reading to learn about 11 Exciting New Features and Changes in iOS 14.2.
New Emojis
iOS 14.2 and iPadOS 14.2 have thirteen new emoji characters, including a smiling face with a tear, a ninja, pinched fingers, an anatomical heart, a bell pepper, and more. There are also new animals such as a black cat, a mammoth, a polar bear, and the much-loved, but now extinct, dodo bird. Including variations, there are over 100 new emoji in total.
New Wallpapers
Apple has eight new wallpapers that include both artistic scenes and realistic natural scenes. There also are new wallpaper choices for dark and light mode.
Updated Apple Watch App
Apple updated the icon for the Apple Watch app to reflect the company's latest Apple Watch Series 6 and Watch SE options. The new Apple Watch icon does away with the typical clasp band and replaces it with the Solo Loop band.
Apple Card History Tracking
Use the Apple Card to make purchases and want to track your yearly spending habits? Well, now you can in iOS 14.2. Previously, users could only view their spending on a weekly or monthly basis. The new interface also lets users track the Daily Cash they've earned on their purchases.
Exciting New Features & New Plans 12x16
Optimized Battery Charging for Apple AirPods
AirPods owners may see improved battery performance over the life of their device thanks to the new optimized battery charging Apple added to iOS 14.2. The feature monitors your usage habits and optimizes the battery charging cycle. The goal is to minimize the wear and tear on the battery and increase available power right when you need it.
iPad Gets A14 Camera Support
All A14-equipped iPad Air owners can now use scene detection, a camera feature that identifies objects in your surroundings to improve the quality of the resulting photo. The update also adds Auto FPS, which will enhance low light videos by reducing the frame rate of the video as it is being recorded.
Exciting New Features & New Plans Floor Plans
People Detection
People detection is a new accessibility feature that uses the camera to judge the distance to another person. It can be helpful for those with eyesight problems to maintain a safe social distance.
Music Recognition
Exciting New Features For Small
Apple added a new music recognition feature to the Control Center. You can turn on Apple's Shazam music recognition feature using a toggle in settings. Once enabled, music recognition is available all the time from the control center. You can simply open the control center and tap the Shazam icon to fire up music recognition.
Now Playing Widget and AirPlay Interface
The newly refreshed 'Now Playing' widget in the Control Center now lists recently played albums. This list appears when there is no music playing, giving you the chance to revisit some of your most recent tunes. Also, a new AirPlay interface lets you easily select multiple AirPlay 2 compatible devices at the same time.
Exciting New Features For Ipad
Personal Update
Personal Update is a new Siri feature similar to the daily briefing Amazon added to its Echo devices. The personal update feature gathers the information that is relevant to you and compiles it into a short minute or two blurb. The news bulletin can include the local weather, your calendar events, and other relevant news. It generates the information using your HomePod speaker and supports the multi-user feature, so a blurb can be tailor-made for a specific user.
Intercom
Exciting New Features & New Plans One Story
Intercom is a new HomePod feature that allows family members to communicate with each other inside a home. Members can send messages through the HomePod speakers to connected iPhones, iPads, Apple Watches, and AirPods. It even sends notifications to CarPlay, so users can receive these voice notes when they are out and about.
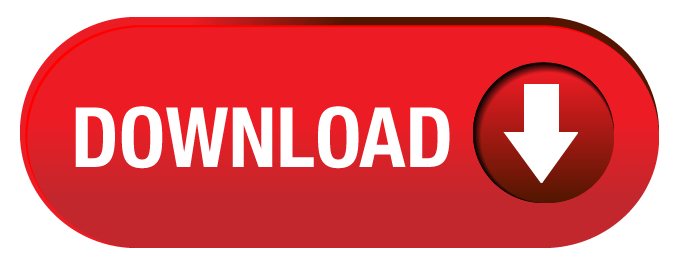